Hi, In this post I’m going to share a little overview of .NET Core and Asp.NET Core. The journey of .NET Core started with the purpose of Microsoft to build a common .NET base library that provides a common baseline that all platforms. With this vision, Microsoft had released the first open-source version of .NET Core 1.0 release on 27 June 2016, along with ASP.NET Core 1.0 and Entity Framework. This ASP.NET Core is a redesigned version of ASP.NET that can run on .NET Core or .NET Framework both. The developers can use ASP.NET Core to build web apps and services, IoT apps, and mobile backends. ASP.NET Core is also available on three different platforms — Windows, OS X, and Linux.
ASP.NET Core cross-platform, high-performance, open-source framework for building web and cloud applications. Through ASP.NET Core, we can develop web apps and services, IoT apps, and mobile backends by using our favorite development tools on Windows, macOS, and Linux.
Prerequisites
-
First, you need to download Dotnet core 3.0 if you don't have it. Download recommended version from here: https://dotnet.microsoft.com/download/dotnet-core
-
Visual Studio 2019, You can download community version from here: https://visualstudio.microsoft.com/vs/
Getting started with Core 3.0 web API
Step 1:- Open Visual Studio 2019 on your computer.
Step 2:- Now click at "Create a new Project" area in Visual Studio 2019.
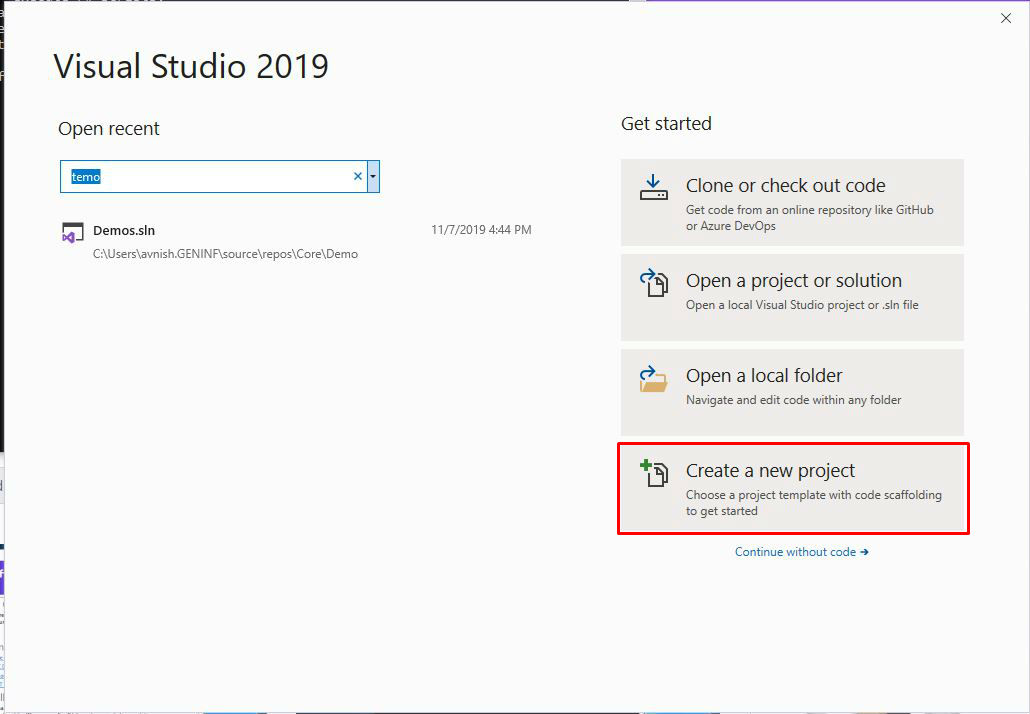
Step 3:- Select "ASP.NET Core Web Application" and hit at Next Button.
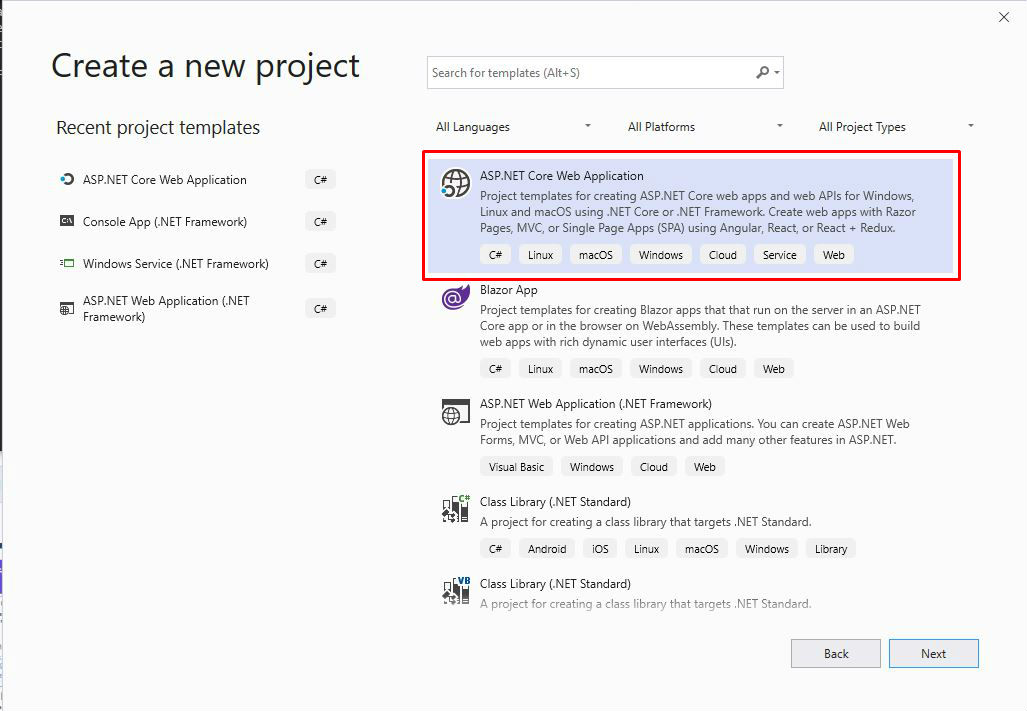
Step 4:- In the next window, fill your project details and location. Then hit at Create Button.
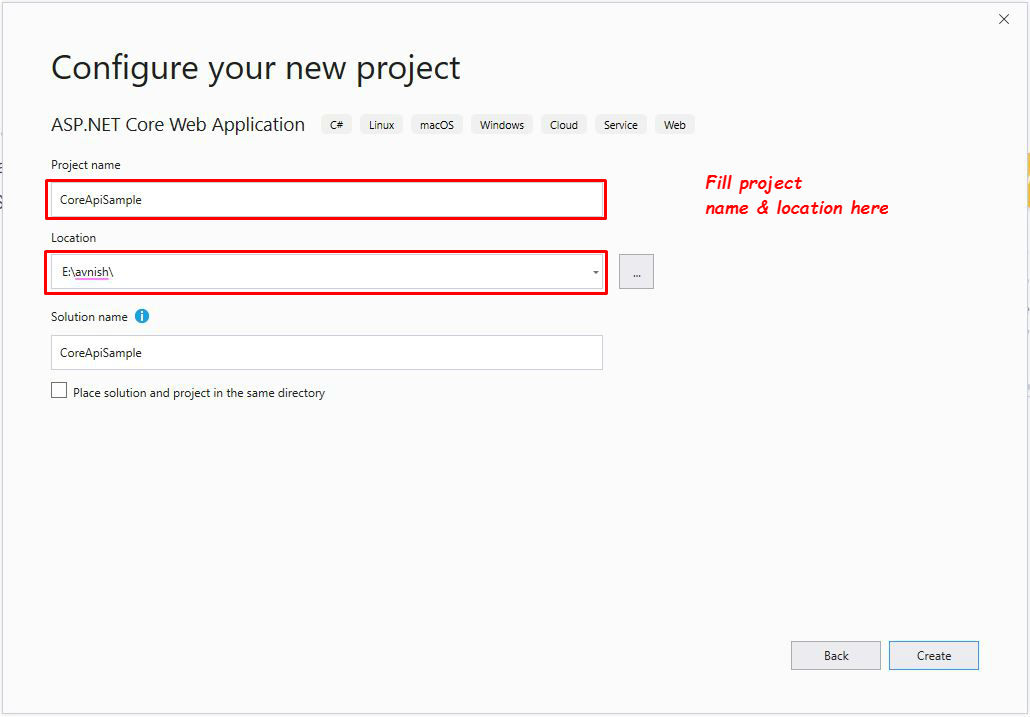
Step 5:- So here the Api Template Selection UI shows up. Before selection, you should have a look at two dropdowns on top. Make sure those are filled accordingly below the image. Now select the API as your project type and click Create Button.
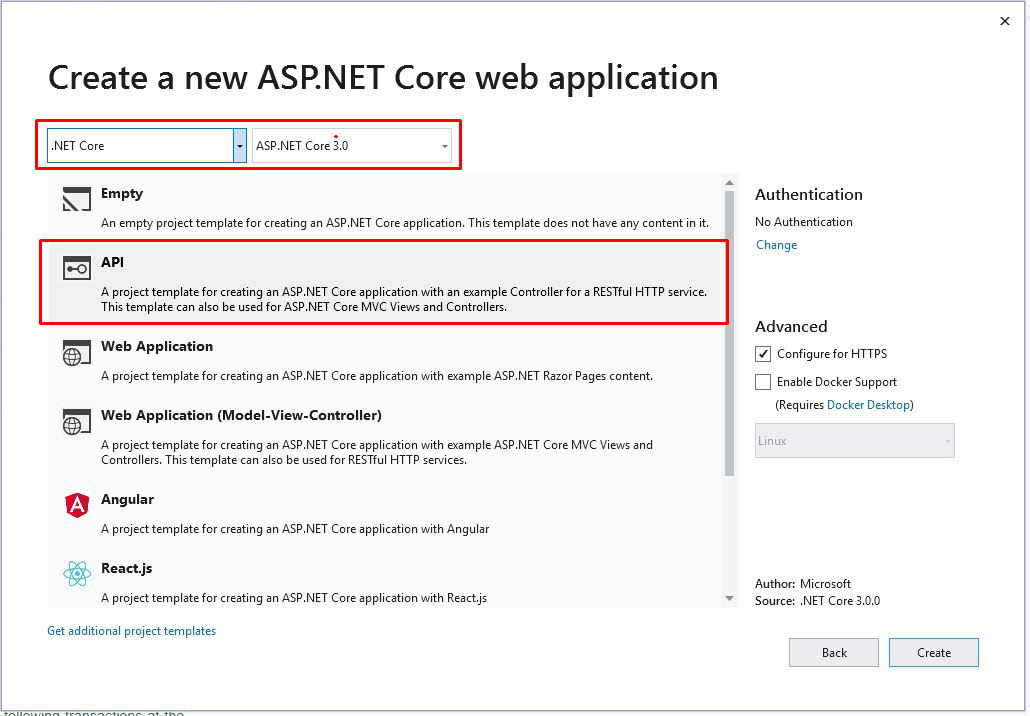
Step 6:- After some loading, this project loads and you can see in the solution explorer of your visual studio with proper project structure. you can build, run and test the project.
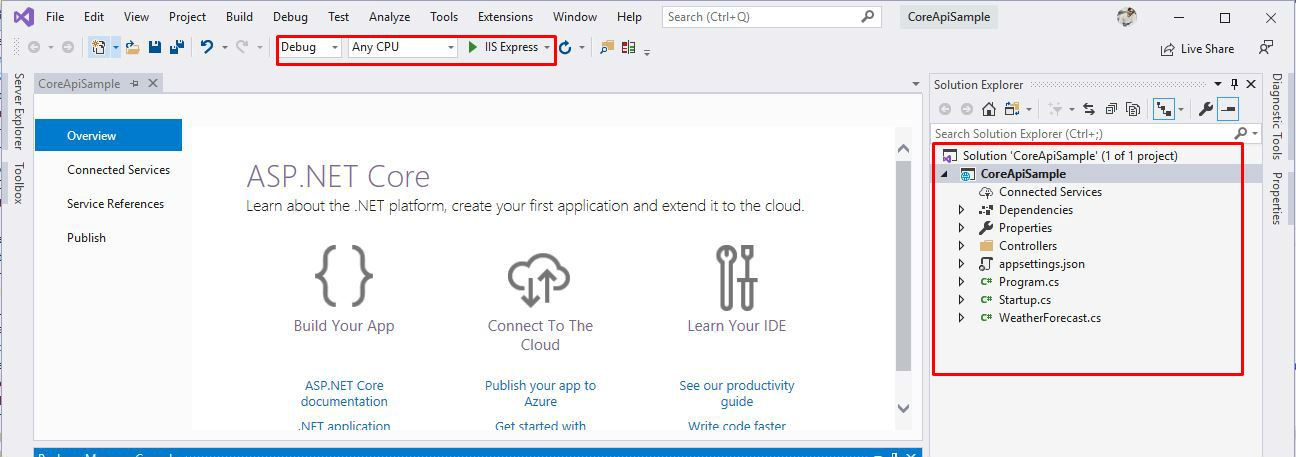
Step 7:- If all looks good, its time to EF core in the project. EF Core does not support visual designer for the DB model and wizard to create the entity and context classes similar to EF 6, So for Adding EF Core, there are two possibilities.
1. Database First (With existing Database)
2. Code First (With a new Database)
EF core Database First Approach:- If you have an existing database, using the Scaffold-DbContext command, you can generate entity and context classes based on the schema of the existing database. For the same go step by step.
1. Open Package Manager Console(From the View menu, select Other Windows > Package Manager console. )
2. Install below the NuGet package one by one.
> Install-Package Microsoft.EntityFrameworkCore.SqlServer -Version 3.0.0
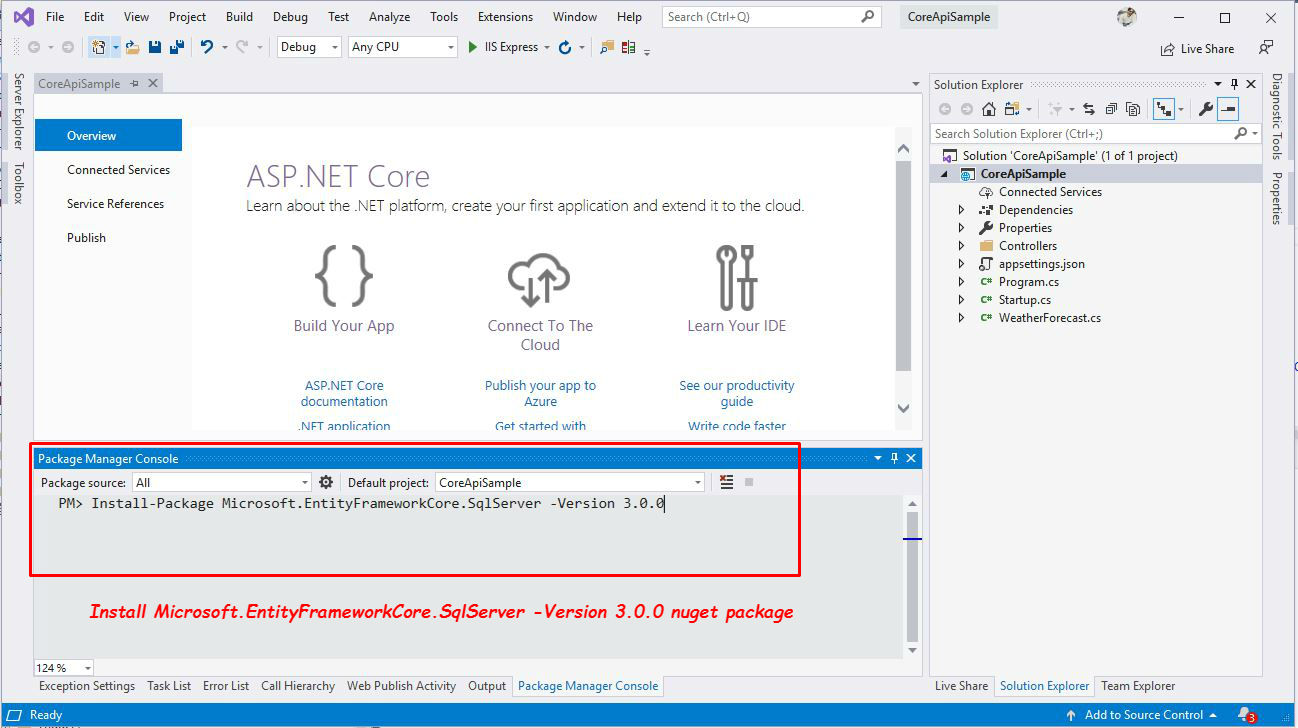
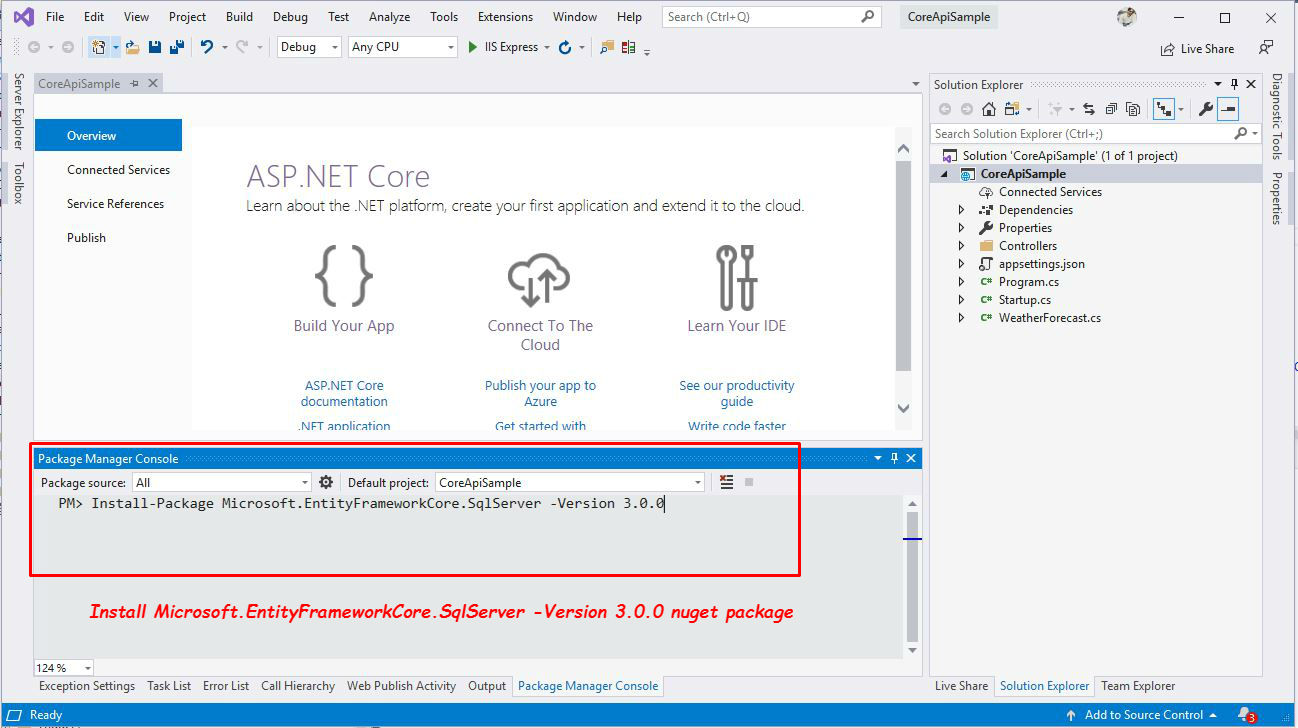
> Install-Package Microsoft.EntityFrameworkCore.Design -Version 3.0.0
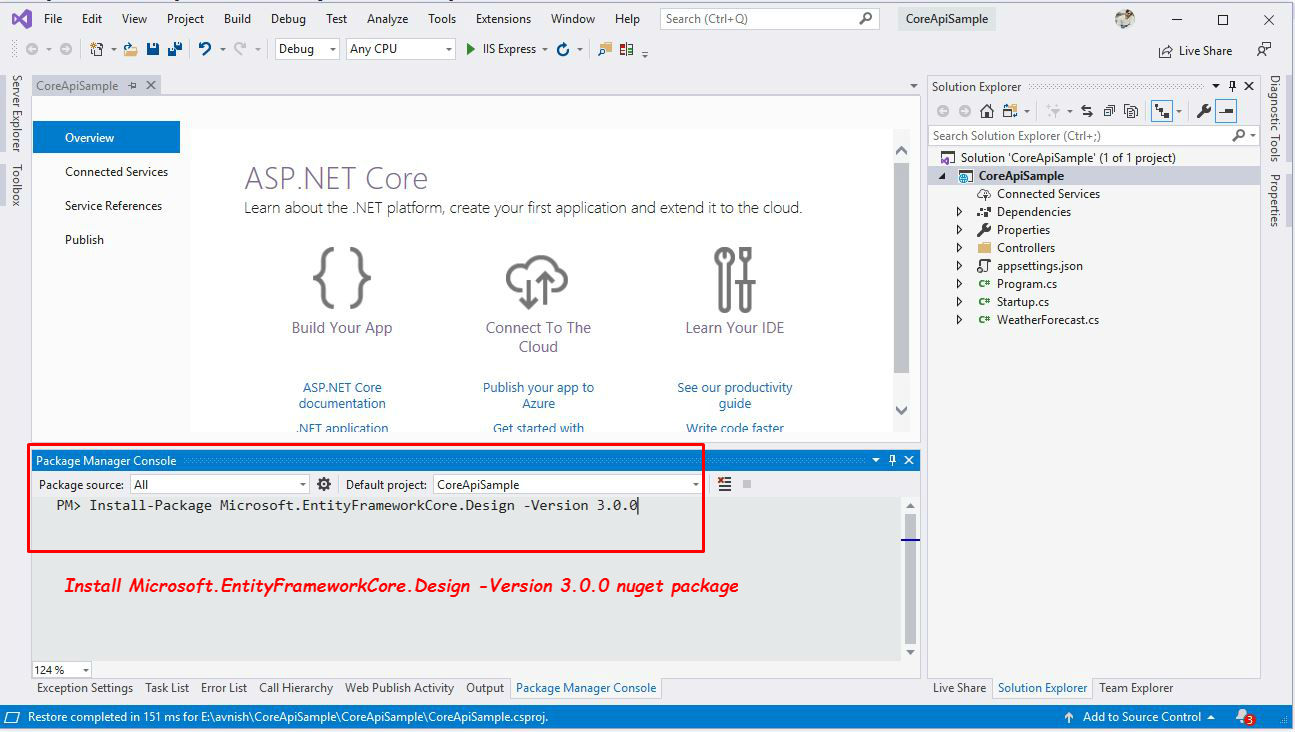
> Install-Package Microsoft.EntityFrameworkCore.Tools -Version 3.0.0
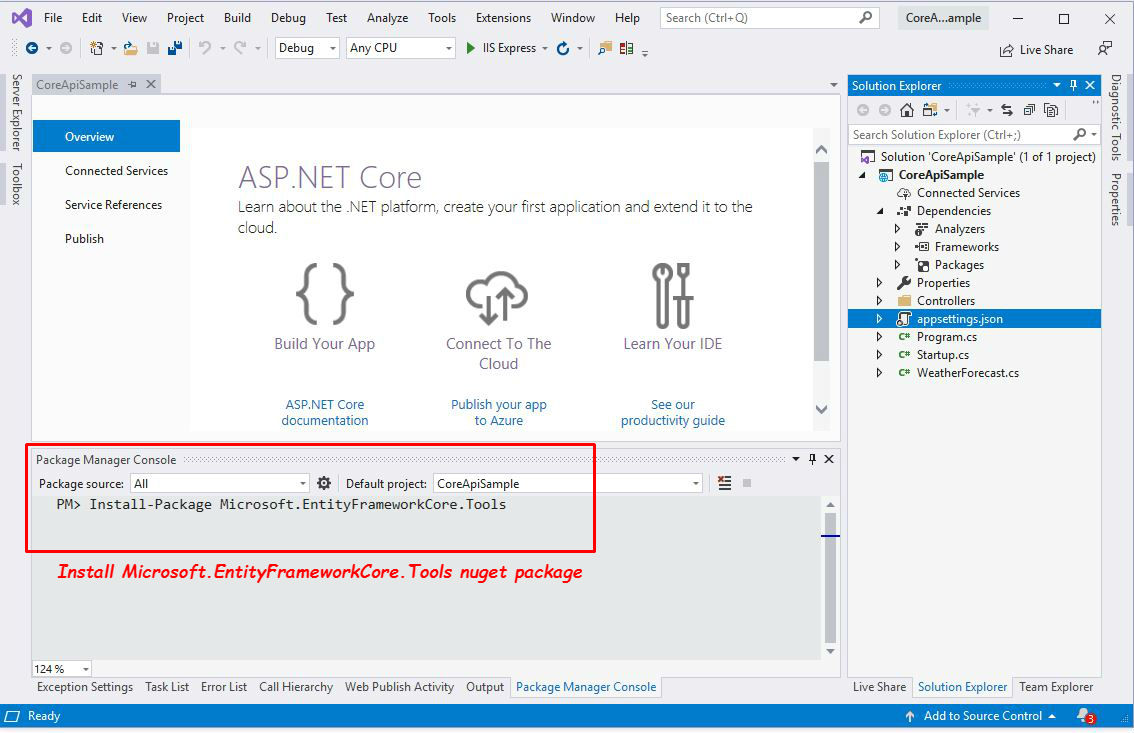
3. Now time to generate entity and context classes, run following command in Package Manager console.
Scaffold-DbContext "Server=.;Database=CoreApi;Trusted_Connection=True;" Microsoft.EntityFrameworkCore.SqlServer -OutputDir Models -Context CoreDbContext -DataAnnotations
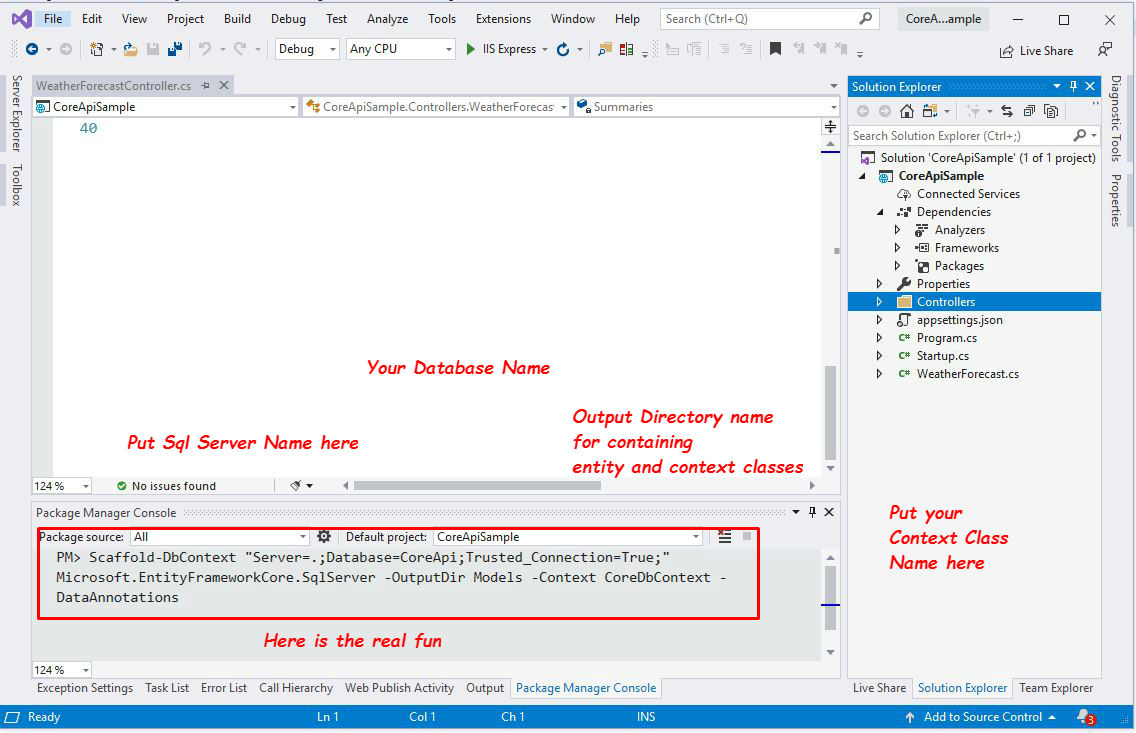
---------------OR---------------
EF core Code First Approach:- You need to create the class that derives from DbContext, aka context class. This context class typically includes DbSet properties for each entity in the model.
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
namespace CoreApiSample.Models
{
public partial class Classes
{
public Classes()
{
Questions = new HashSet();
}
[Key]
public Guid Id { get; set; }
public DateTimeOffset Created { get; set; }
[StringLength(255)]
public string CreatedBy { get; set; }
public DateTimeOffset? Modified { get; set; }
[StringLength(255)]
public string ModifiedBy { get; set; }
public bool IsActive { get; set; }
[Required]
[StringLength(50)]
public string Name { get; set; }
[Required]
[StringLength(50)]
public string Abbrasive { get; set; }
[InverseProperty("Class")]
public virtual ICollection Questions { get; set; }
}
}
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
namespace CoreApiSample.Models
{
public partial class Questions
{
[Key]
public Guid Id { get; set; }
public DateTimeOffset Created { get; set; }
[StringLength(255)]
public string CreatedBy { get; set; }
public DateTimeOffset? Modified { get; set; }
[StringLength(255)]
public string ModifiedBy { get; set; }
public bool IsActive { get; set; }
public Guid? ClassId { get; set; }
public Guid? SubjectId { get; set; }
[Required]
public string QuestionsText { get; set; }
public string SolutionText { get; set; }
public byte[] ImageSolution { get; set; }
[StringLength(20)]
public string ImageMimeType { get; set; }
[Column("VideoSolutionURL")]
[StringLength(500)]
public string VideoSolutionUrl { get; set; }
public string Tag { get; set; }
[StringLength(50)]
public string Writer { get; set; }
[StringLength(50)]
public string Book { get; set; }
[ForeignKey(nameof(ClassId))]
[InverseProperty(nameof(Classes.Questions))]
public virtual Classes Class { get; set; }
[ForeignKey(nameof(SubjectId))]
[InverseProperty(nameof(Subjects.Questions))]
public virtual Subjects Subject { get; set; }
}
}
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
namespace CoreApiSample.Models
{
public partial class Subjects
{
public Subjects()
{
Questions = new HashSet();
}
[Key]
public Guid Id { get; set; }
public DateTimeOffset Created { get; set; }
[StringLength(255)]
public string CreatedBy { get; set; }
public DateTimeOffset? Modified { get; set; }
[StringLength(255)]
public string ModifiedBy { get; set; }
public bool IsActive { get; set; }
[Required]
[StringLength(50)]
public string Name { get; set; }
[Required]
[StringLength(50)]
public string Abbrasive { get; set; }
[InverseProperty("Subject")]
public virtual ICollection Questions { get; set; }
}
}
using System;
using Microsoft.EntityFrameworkCore;
using Microsoft.EntityFrameworkCore.Metadata;
namespace CoreApiSample.Models
{
public partial class CoreDbContext : DbContext
{
public CoreDbContext()
{
}
public CoreDbContext(DbContextOptions options)
: base(options)
{
}
public virtual DbSet Classes { get; set; }
public virtual DbSet Questions { get; set; }
public virtual DbSet Subjects { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
if (!optionsBuilder.IsConfigured)
{
optionsBuilder.UseSqlServer("Server=.;Database=CoreApi;Trusted_Connection=True;");
}
}
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity(entity =>
{
entity.Property(e => e.Id).ValueGeneratedNever();
entity.Property(e => e.Abbrasive).IsUnicode(false);
entity.Property(e => e.Name).IsUnicode(false);
});
modelBuilder.Entity(entity =>
{
entity.Property(e => e.Id).ValueGeneratedNever();
entity.Property(e => e.Book).IsUnicode(false);
entity.Property(e => e.ImageMimeType).IsUnicode(false);
entity.Property(e => e.Tag).IsUnicode(false);
entity.Property(e => e.Writer).IsUnicode(false);
});
modelBuilder.Entity(entity =>
{
entity.Property(e => e.Id).ValueGeneratedNever();
entity.Property(e => e.Abbrasive).IsUnicode(false);
entity.Property(e => e.Name).IsUnicode(false);
});
OnModelCreatingPartial(modelBuilder);
}
partial void OnModelCreatingPartial(ModelBuilder modelBuilder);
}
}
Step 8:- Now time add the Connection string at your appsettings.json. Add below lines to appsettings.json.
"ConnectionStrings": {
"Database": "Server=(local);Database=CoreApi;Trusted_Connection=True;"
},
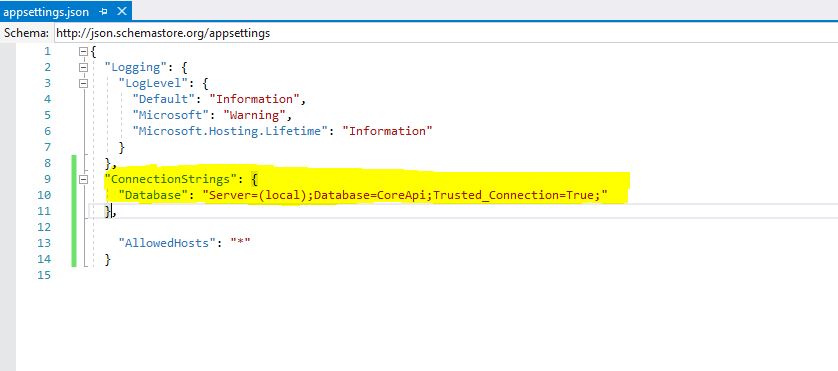
Step 9:- Now time add the DbContext at your Startup.cs. Add below lines to Startup.cs.
services.AddDbContext(op => op.UseSqlServer(Configuration.GetConnectionString("Database")));
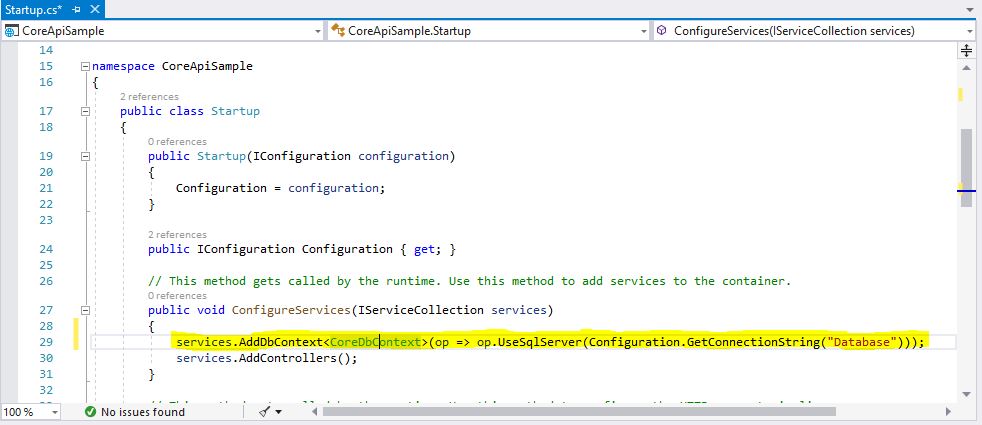
Step 10:- If You are using Code First Approach, then you have to run the Migration commands in the Package Manager Console. For the same go step by step.
1. Open Package Manager Console(From the View menu, select Other Windows > Package Manager console. )
2. Run below command one by one.
> Add-Migration CreateDb
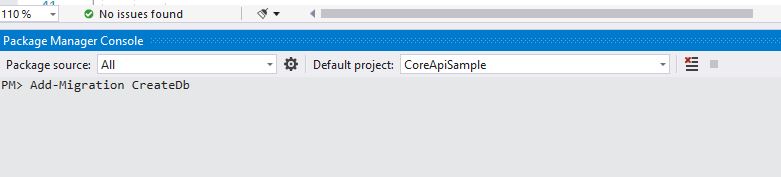
> Update-Database
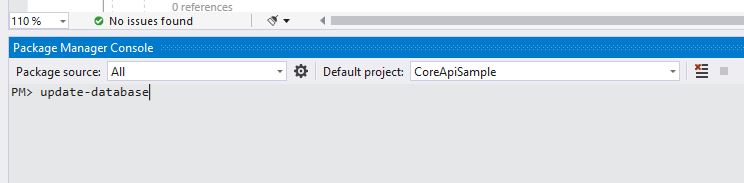
Step 10:- Now, Your web API is ready to build and run. Waiting for what runs it
.

Wrapping up
In this post we’ve learned how to we can create Asp.net core 3.0 web API using EF Core. In the future post, I will let you know the implementation of Swagger documentation in the same API Project. If you like this article feel free to share it with your friends.